This tutorial is to make you familiar with the Google Captcha it is known as Google re-captcha, very useful plugin or say api to prevent the spamming on your comment forms, this tutorial makes use of PHP and ajax
How to use google recaptcha with PHP and Javascript AJAX
Posted on Monday 15th December 2014. ยท 1437 Views Share
This tutorial is to make you familer with the Google Captcha it is known as Google re-captcha, very useful plugin or say api to prevent the spaming on your comment forms, this tutorial makes use of PHP and ajax
You may have seen a captcha on many web forms, Captcha is a way to avoid the spamming via the bots. Captcha uses the image with text or numbers which is somewhat distorted. User can understand but is not possible for the bot to make the text corrosponding to that Image but human can understand and fillup the form.
One of the same is google’s recaptcha which is available with various language, here I will show the example with the php, integration of this. first of all you need to register your website with the recaptcha webpage here.
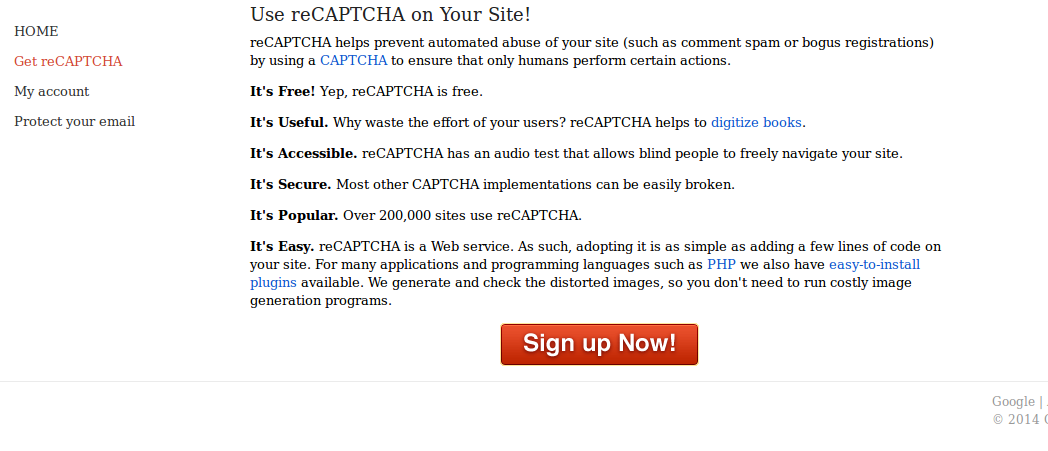
Next you need to register the domain, to get a unique private and public key for your website. You can check the option to use the key for multiple domains.
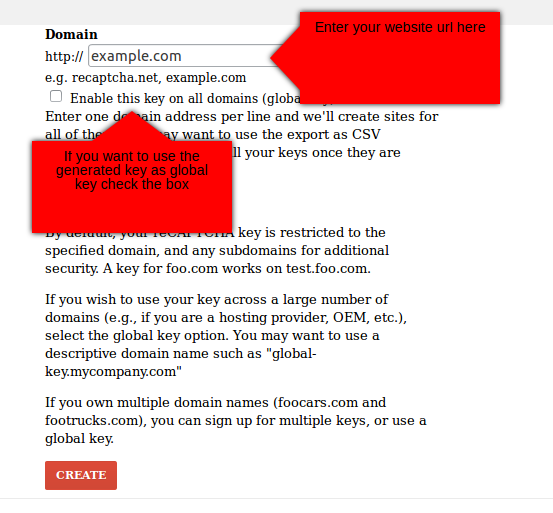
This will give you two keys a public key and a private key, keep them safe you will need these. As I am going to demonstrate this with php so I am going to use php plugin library. you can find all the libraries and plugins here.
We are nearly set with the registration and prepration, lets do some coding now.
I am creating 3 files,
testcaptcha.php – client side captcha implementation
verify.php – server side captcha verification script
demo.php – this is nothing but the final outcome, or you can say the place where form will be submitted.
fourth is recaptchalib.php which is not created by me but is the actual google captcha plugin file.
first of all lets create a client side page, I am creating nothing but only placing the captcha plugin on this page, here is the complete code
<!--testcaptcha.php--> <html> <head> <script src="//ajax.googleapis.com/ajax/libs/jquery/1.10.2/jquery.min.js"></script> <script type="text/javascript"> function validate(){ var rcf = $("#recaptcha_challenge_field").val(); var rrf = $("#recaptcha_response_field").val(); $.post("verify.php",{recaptcha_challenge_field:rcf, recaptcha_response_field:rrf},function(data){ if(data.trim()=="true"){ $("#captcha").submit(); } else{ $("#cpmsg").html("Incorrect CAPTCHA! Please try again"); Recaptcha.reload(); } }); } </script> </head> <body> <!-- the body tag is required or the CAPTCHA may not show on some browsers --> <!-- your HTML content --> <?php require_once('recaptchalib.php'); $publickey = "your public key goes here"; // you got this from the signup page echo recaptcha_get_html($publickey); ?> <form method="post" id="captcha" action="done.php"> <input type="button" onclick="validate();" /> <div id="cpmsg"></div> </form> <!-- more of your HTML content --> </body> </html>
Don’t forget to replace public key variable value with your own public key.
You may have noticed that I have used two id’s to fetch values, but the id’s does not exists anywhere on this file. Well, you don’t need to worry about that, these are the fields generated by the captcha library on the page.
I have used the jQuery post method to reduce my work please feel free to do whatever change you like to do.
second file is the file I have used to verify the correction of captcha this is a server side file and you will use your private key in there. the file is like this
<?php //file name: verify.php require_once('recaptchalib.php'); $privatekey = "place your private key here"; $resp = recaptcha_check_answer ($privatekey, $_SERVER["REMOTE_ADDR"], $_POST["recaptcha_challenge_field"], $_POST["recaptcha_response_field"]); if (!$resp->is_valid) { // What happens when the CAPTCHA was entered incorrectly die ("The reCAPTCHA wasn't entered correctly. Go back and try it again." . "(reCAPTCHA said: " . $resp->error . ")"); } else { // Your code here to handle a successful verification echo "true"; } ?>
Again don’t forget to replace the “your private key here” with your actual private key.
This is simple php I don’t think I need to explain much about this, rather you will get this file as it is from the recaptcha page.
finally last file done.php, your contol will finally move to this file when the captcha is verified.
<?php echo "hello world"; ?>
I have used jquery to submit the form, this is actually because I am using ajax to verify, If you want to use the serverside verification you don’t need to do much, you can just place the captcha script inside the form element and on submit it will be verified.
Reference:
References: Google Recaptcha