JSP, Servlet Context Initialization and Request Parameters, allows you to initialize the variables on startup in a global scope and get form or url parameters which are send via form submission or via dynamic url creation.
In this article we are going to explain some key points of the servlet and JSPs. Now what is a JSP we never discussed it before, right? Yes, We are going to introduce the JSP in this article. However the scope will be limited to the introduction on JSP only.
What is JSP?
JSP are Java Server Pages. That means when you are working with web application in java, JSP give you more flexibility and code reduction. Because with JSP you put java in HTML instead of opposite. But you need not to worry as this gives you more power, does not take away the power from you. The JSP is ultimately converted into a server when a jsp page is first invoked by the user. When next request arrives it is served by the servlet that was generated by first request. This saves the time for request.
The JSP provide all the flexibility you get with the servlets, and even more. You can combine the JSP and servlet to make a better out of your application. there are some tags which we know as scriptlets. these scriptlets help to perform the java operations between the HTML code. This looks inspired by the scripting languages like php, but yes this is helpful.
There are three type of scriptlets which are key for the development however there are more for providing the helper functionality.
<%! %> - used for the variable declaration <% %> - used for language structure and flow control coding <%= %> - used for the variable output
Beside this the JSP provide pre defined variables request, response and out which serves as the reference for the HttpServletRequest, HttpServletResponse and PrintWriter respectively, so you don’t have to bother about them either.
However before we start up with the JSP and the parameter passing through the request to servlet, we will talk about the Initialization parameters, which we can do using ServletContext.
The servletContext is a parameter scope which lets you define the parameter using the web.xml. This parameter is a name-value pair which can be accessed anywhere in the application scope. We define it in the web.xml file which is known as deploy descriptor. You can access these parameters in the servlet using the method, getServletContext. We will present the code in a moment.
<?xml version="1.0" encoding="UTF-8"?> <web-app version="3.1" xmlns="http://xmlns.jcp.org/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd"> <servlet> <servlet-name>HelloWorldServlet</servlet-name> <servlet-class>home.HelloWorldServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>HelloWorldServlet</servlet-name> <url-pattern>/HelloWorldServlet</url-pattern> </servlet-mapping> <session-config> <session-timeout> 30 </session-timeout> </session-config> <context-param> <param-name>website</param-name> <param-value>www.examsmyantra.com</param-value> </context-param> </web-app>
This is how we define the parameter in the web.xml, in the context-param tag we define the param-name and value and close it. Now this value can be accessed in the application scope anywhere using the servlet context. As we show it in the following servlet program
package home; import java.io.IOException; import java.io.PrintWriter; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import javax.servlet.ServletConfig; import javax.servlet.ServletContext; public class HelloWorldServlet extends HttpServlet { PrintWriter out; @Override protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { out = response.getWriter(); ServletContext sc = getServletConfig().getServletContext(); out.println(sc.getInitParameter("website")); } }
We first get the servlet Context using the servlet config, then use this to get the parameter. Servlet Context provide the method by which we can access the value based on the name. There is another method getInitParameterNames() which returns the Names in the form of Enumeration.
The running of servlet produces following output.
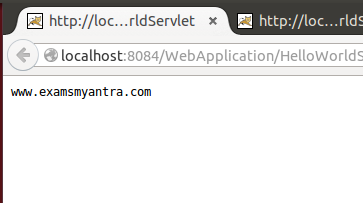
Now that we are familiar with the servlet Context lets move on and see how we can work with the JSP page and the parameters we pass through the URL or Form submission.
First we are going to make a JSP, which will initialize a variable and use that variable on the page, and do a sample looping. Also we are going to create a form on the same page and we will modify out Hello World Servlet to receive parameters from this JSP and display them on screen.
<%@page contentType="text/html" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>JSP Page</title> </head> <body> <%! String s = "ExamsMyantra"; %> <h1>Please Enter your details to <%= s %></h1> <form action="HelloWorldServlet" method="post"> <div><input type="text" name="name"></div> <div><input type="text" name="email"></div> <div><input type="submit" value="submit"></div> </form> <% for(int i=0; i<5; i++){ out.println(i); } %> </body> </html>
Now in this JSP we are creating or say defining a variable of type String, which contains value ExamsMyantra, and we will simply Print it as a part of Form Heading using the Other scriptlet. Finally we create a form which will submit to HelloWorldServlet and a for loop which will just print the value 0 to 5 on page. This is just a demo page to show you the working and structure of the JSP page.
On the Top of this page you must have noticed we have another type of scriptlet, <%@ %> these are used to define the properties of the web page. and any Import statements are also included on these.
This page looks like this when run:
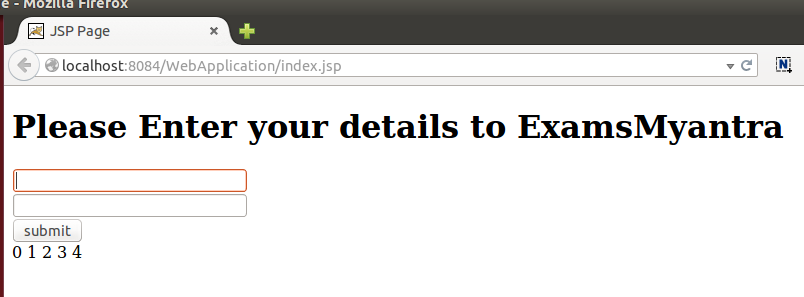
and here is the servlet which will handle the request from the jsp page.
package home; import java.io.IOException; import java.io.PrintWriter; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import javax.servlet.ServletConfig; import javax.servlet.ServletContext; public class HelloWorldServlet extends HttpServlet { PrintWriter out; protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException{ out = response.getWriter(); out.println("The Name is"+request.getParameter("name")); out.println("The email is"+request.getParameter("email")); } }
In this as we are passing the form values using post method we are going to use the doPost method. The request reference provide us a method getParameter(String param-name); using this method we can get the form field values. All you need to do is to invoke the method on the request reference with the name of form field as a parameter to the method. The result looks like this.
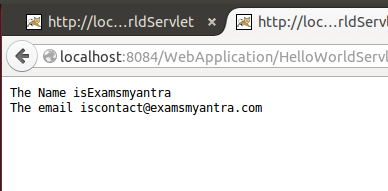
We hope that this was easy enough to understand, Again We would like to mention that this article is intented for beginners and is limited in scope. So please read official documentation for the details about the servlet or JSP.